Learn Multi platform 8086 Assembly
Programming... For World Domination!
Simple Samples
In this series we'll look at simple tasks...
each will be a single ASM file, and will compile into a usable example you
can build on
 |
Lesson
S1 - Sprite drawing and Simple key movement in MS DOS
Lets learn how to create a sprite, and move it around the screen
with the keyboard. |
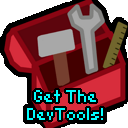 |
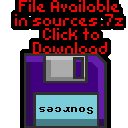
Dos_Keyboard.asm |
|
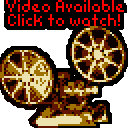 |
Starting our program
Our program starts with the basic definitions of a SMALL
program, and a STACK size.
We then use INT 10h to turn on the bitmap screen, we're using 256
color mode, so each pixel will be 1 byte |
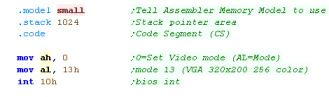 |
Drawing a sprite
We're going to move a 8x8 smiley around the screen |
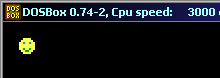 |
You can use my Akusprite Editor to create files in the valid
format for the DOS screen. It's free and open source - so you have
no excuse!
You can learn more about AkuSprite Editor here. |
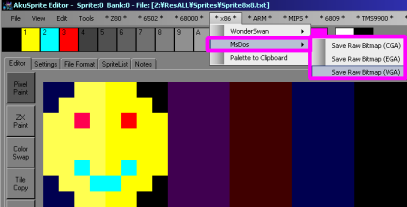 |
Our Sprite is included in the source code |
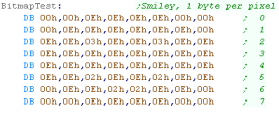 |
We pass an X,Y pos in DH,DL
Each line is 320 pixels (320 bytes)... we move in 8x8 'blocks' so
we multiply the Xpos by 8, and they Ypos by 320*8
We then add the screen base - 0A000h:0000h |
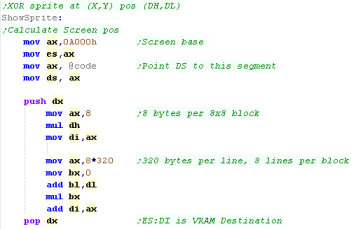 |
We 'XOR' the sprite with the screen pixels... this 'inverts' the
image on the screen, meaning if it's drawn twice to the same pixel
position it will be removed the second time. |
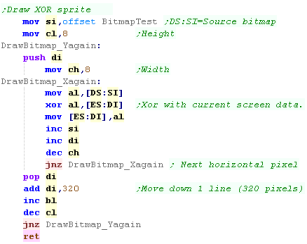 |
Key reading and moving the sprite
We define the starting position of the sprite, and show the
starting smiley position with our showsprite routine. |
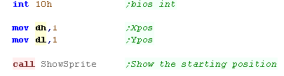 |
We need to process the 4 directions.
We use INT 16h - Function 01 to read the keyboard, this returns NZ
if a key is waiting in the key buffer.
if there is then first we remove the old sprite, then we use INT
16h - Function 00 to get the keycode in AH
If a direction key is pressed, we then check the current smiley
position - if it's already at the edge of the screen, we can't
move again, but if we can, we move in the pressed direction.
We repeat for all 4 directions Up, Down, Left and Right. |
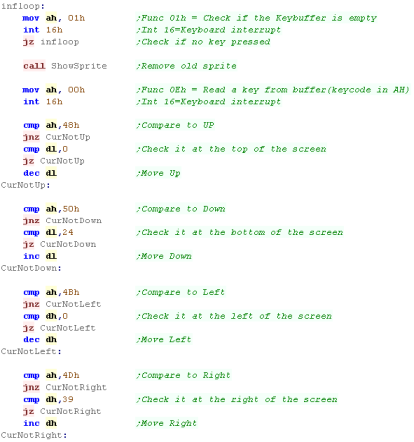 |
We then repeat for any future movements. |
 |

 |
Lesson
S2 - Sprite drawing and Simple key movement on the Wonderswan
Lets write a sprite moving example on the Wonderswan and
Wonderswan color.
The wonderswan is 4 color, and the WSC is 16 color, so the
examples will be different |
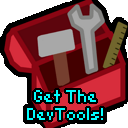 |
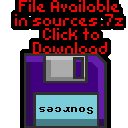 |
|
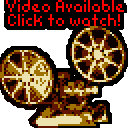 |
Starting our program
When our program starts, we need to define a Stack pointer |
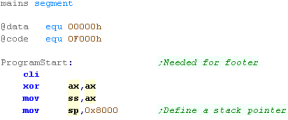 |
next we need to enable the graphics screen.
We're using a tilemap mode.
The settings are slightly different on the Wonderswan Color |
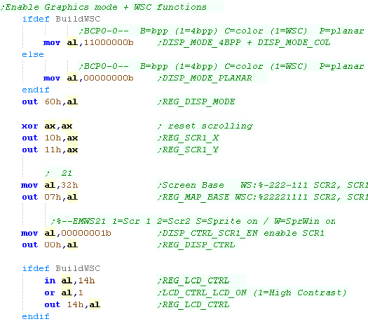 |
We need to set up a palette...
On the Wonderswan we define 8 greyshades, and select 4 for our
palette
On the Wonderswan Color we define 16 colors for our palette |
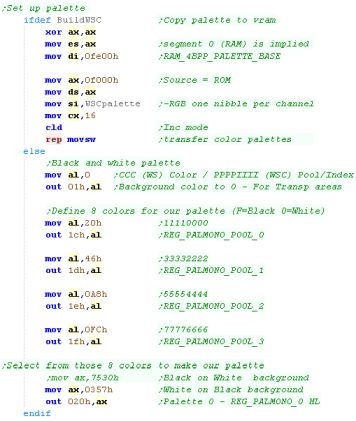
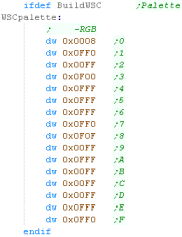 |
We'll clear the tilemap (at 0000:1000+) with tile 0
The tilemap is 32x32 tiles |
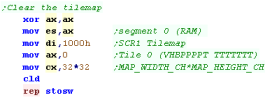 |
We need to transfer our smiley sprite to VRAM.
The Vram address is 0000:2000 on the Wonderswan
The Vram address is 0000:4000 on the Wonderswan Color |
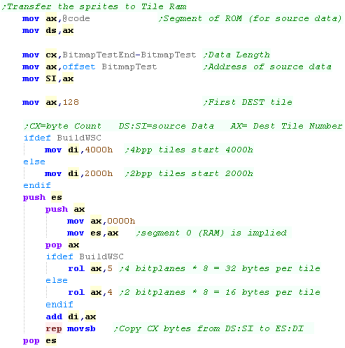 |
Drawing a sprite
We're going to move a 8x8 smiley around the screen |
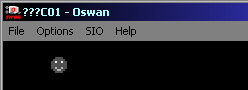
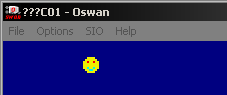 |
You can use my Akusprite Editor to create files in the valid
format for the DOS screen. It's free and open source - so you
have no excuse!
You can learn more about AkuSprite Editor here. |
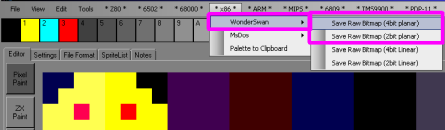 |
Our Sprite is included in the source code |
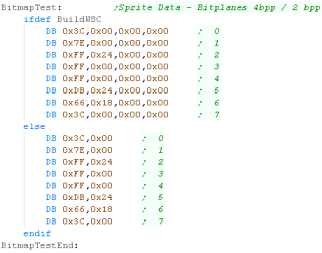 |
Lets define a tile on screen!
The tilenumber is passed in AL
We pass an X,Y pos in DH,DL
Each line of the tilemap is 32 tiles.. each tile is 2 bytes...
the Tilemap base is 0000h:1000h
so our formula for the tile to change is 1000h + (Xpos * 2) +
(Ypos * 64) |
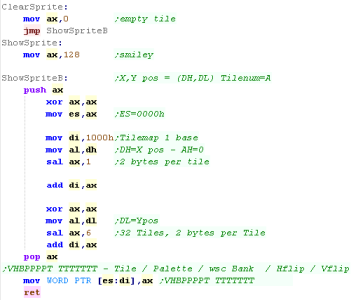 |
Pad reading and moving the sprite
We define the starting position of the sprite, and show the
starting smiley position with our showsprite routine. |
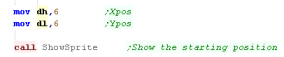 |
We need to process the 4 directions.
we select one of the 3 sets of controls (X-Pad, Y-Pad buttons)
by writing to 0B5h with one of the top 4 bits set.
We then read from 0B5h to get the current state of those buttons
in the bottom 4 bits.
if a key is pressed we first remove the old sprite
We check if each direction key is pressed, we then check the
current smiley position - if it's already at the edge of the
screen, we can't move again, but if we can, we move in the
pressed direction.
We repeat for all 4 directions Up, Down, Left and Right. |
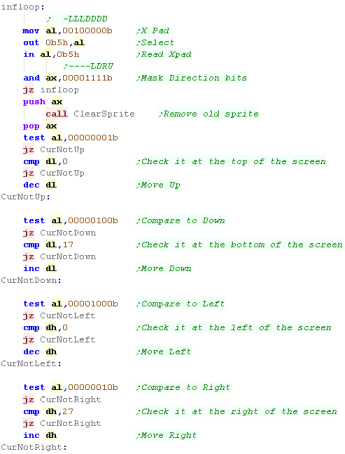 |
We now draw the new sprite position, pause a moment and
repeat. |
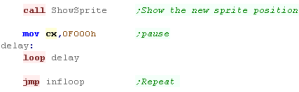 |
| |
Buy my Assembly programming book on Amazon in Print or Kindle!
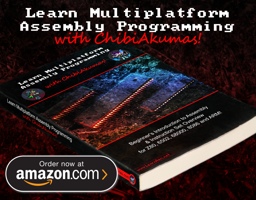
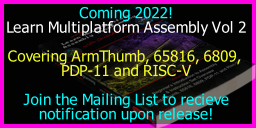
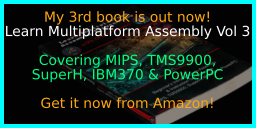
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
|